■gb.display.drawRect関数
Gamebuimo.hライブラリのgb.display.drawRect関数は、指定された場所に四角形を描画します。
使用される色は、gb.display.setColor関数を使用して定義されます。
四角形の一部が画面外になるような値を指定すると、レンダリングされません。
■使用例
gb.display.drawRect関数を使ったArduinoIDEのプログラム例は図1の通りです。
このプログラムを実行するとsetup関数中のgb.begin関数でGamebuinoオブジェクトを初期化し、gb.titleScreen関数で画面に「DYNAMIC TILE MAP DEMO」と表示します。
スタート画面でキーボードの「K」(GamebuinoのA)ボタンを押すと画面上にビットマップ図形がタイル状に表示され、gb.display.drawRect関数によって現在選択されているタイル上に四角形の枠を表示します。
参考に図1プログラムをHEXファイルに変換し、それをSimbuino4Webでエミュレートした結果を図2に示します。

#include <SPI.h> #include <Gamebuino.h> Gamebuino gb; const byte grass[] PROGMEM = {16, 16, 0x10, 0x0, 0x28, 0x2, 0x10, 0x0, 0x0, 0x0, 0x0, 0x0, 0x10, 0x40, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x80, 0x0, 0x0, 0x40, 0x0, 0x0, 0x0, 0x0, 0x8, 0x4, 0x0, 0x0, 0x0,}; const byte rocks[] PROGMEM = {16, 16, 0x20, 0x0, 0x50, 0x0, 0x21, 0xe1, 0x6, 0x18, 0x8, 0xc, 0x8, 0x4, 0x3c, 0x6, 0x42, 0x7, 0x40, 0x37, 0x40, 0x1f, 0x23, 0x9e, 0x1c, 0x7e, 0x8, 0x3c, 0x8, 0x78, 0x7, 0xe0, 0x0, 0x0,}; const byte road_straight[] PROGMEM = {16, 16, 0x1f, 0xf8, 0x1f, 0xf8, 0x5e, 0x78, 0x1e, 0x78, 0x1e, 0x78, 0x1e, 0x78, 0x1e, 0x79, 0x1e, 0x78, 0x1f, 0xf8, 0x1f, 0xf8, 0x1e, 0x78, 0x1e, 0x78, 0x9e, 0x78, 0x1e, 0x78, 0x1e, 0x78, 0x1e, 0x78,}; const byte road_turn[] PROGMEM = {16, 16, 0x1f, 0xf8, 0x1f, 0xf8, 0x1f, 0xfc, 0x1f, 0xff, 0x1f, 0xff, 0xf, 0xff, 0xf, 0xff, 0x7, 0xff, 0x87, 0xff, 0x3, 0xff, 0x1, 0xff, 0x0, 0x7f, 0x2, 0x1f, 0x0, 0x0, 0x0, 0x0, 0x40, 0x0,}; #define NUM_SPRITES 4 const byte* sprites[NUM_SPRITES] = {grass, rocks, road_straight, road_turn}; #define WORLD_W 16 #define WORLD_H 8 byte world[WORLD_W][WORLD_H]; byte getSpriteID(byte x, byte y) { return world[x][y] & B00000011; } byte getRotation(byte x, byte y) { return (world[x][y] >> 2) & B00000011; } void setTile(byte x, byte y, byte spriteID, byte rotation) { world[x][y] = (rotation << 2) + spriteID; } int cursor_x, cursor_y; int camera_x, camera_y; void setup() { gb.begin(); initGame(); } void loop() { if (gb.update()) { if (gb.buttons.pressed(BTN_C)) { initGame(); } updateCursor(); drawWorld(); drawCursor(); } } void initGame() { gb.titleScreen(F("DYNAMIC TILE MAP DEMO")); gb.pickRandomSeed(); //pick a different random seed each time for games to be different initWorld(); gb.popup(F("\25:change \26:rotate"), 60); } void initWorld() { for (byte y = 0; y < WORLD_H; y++) { for (byte x = 0; x < WORLD_W; x++) { setTile(x, y, 0, random(0, 4)); } } } void drawWorld() { for (byte y = 0; y < WORLD_H; y++) { for (byte x = 0; x < WORLD_W; x++) { byte spriteID = getSpriteID(x, y); byte rotation = getRotation(x, y); int x_screen = x * 16 - camera_x; int y_screen = y * 16 - camera_y; if (x_screen < -16 || x_screen > LCDWIDTH || y_screen < -16 || y_screen > LCDHEIGHT) { continue; } gb.display.drawBitmap(x_screen, y_screen, sprites[spriteID], rotation, 0); } } } void updateCursor() { byte spriteID = getSpriteID(cursor_x, cursor_y); byte rotation = getRotation(cursor_x, cursor_y); if (gb.buttons.repeat(BTN_A, 4)) { spriteID = (spriteID + 1) % NUM_SPRITES; gb.sound.playOK(); } if (gb.buttons.repeat(BTN_B, 4)) { rotation = (rotation + 1) % 4; gb.sound.playOK(); } setTile(cursor_x, cursor_y, spriteID, rotation); if (gb.buttons.repeat(BTN_RIGHT, 4)) { cursor_x = wrap(cursor_x + 1, WORLD_W); gb.sound.playTick(); } if (gb.buttons.repeat(BTN_LEFT, 4)) { cursor_x = wrap(cursor_x - 1, WORLD_W); gb.sound.playTick(); } if (gb.buttons.repeat(BTN_DOWN, 4)) { cursor_y = wrap(cursor_y + 1, WORLD_H); gb.sound.playTick(); } if (gb.buttons.repeat(BTN_UP, 4)) { cursor_y = wrap(cursor_y - 1, WORLD_H); gb.sound.playTick(); } int camera_x_target = cursor_x * 16 - LCDWIDTH / 2 + 8; int camera_y_target = cursor_y * 16 - LCDHEIGHT / 2 + 8; camera_x = (camera_x * 3 + camera_x_target) / 4; camera_y = (camera_y * 3 + camera_y_target) / 4; } void drawCursor() { int x_screen = cursor_x * 16 - camera_x; int y_screen = cursor_y * 16 - camera_y; if (!(x_screen < -16 || x_screen > LCDWIDTH || y_screen < -16 || y_screen > LCDHEIGHT)) { gb.display.drawRect(x_screen, y_screen, 16, 16); } gb.display.print(F("X")); gb.display.print(cursor_x); gb.display.print(F(" Y")); gb.display.print(cursor_y); byte spriteID = getSpriteID(cursor_x, cursor_y); gb.display.print(F(" I")); gb.display.print(spriteID); byte rotation = getRotation(cursor_x, cursor_y); gb.display.print(F(" R")); gb.display.print(rotation); }
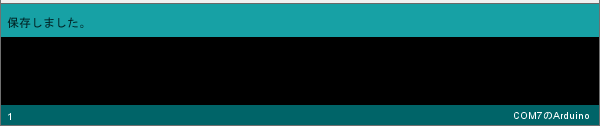
図1:プログラム例
図2:プログラム実行結果
■構文
gb.display.drawRect(x,y,w,h)
■パラメータ
x:左上隅の水平座標。
y:左上隅の垂直座標。
w:矩形の幅。
h:長方形の高さ。
■戻り値
ありません。
励みになりますのでよければクリック下さい(^o^)/