■gb.display.persistence変数
Gamebuimo.hライブラリのgb.display.persistence変数は、各フレーム間で画面を自動的にクリアするかどうかを選択します。
■使用例
gb.display.persistence変数を使ったArduinoIDEのプログラム例は図1の通りです。
このプログラムを実行するとsetup関数中のgb.begin関数でGamebuinoオブジェクトを初期化し、gb.titleScreen関数で画面に「Conway's game of life」と表示します。
スタート画面でキーボードの「K」(GamebuinoのA)ボタンを押すと画面左上にランダムな図形が表示され、reset関数中でgb.display.persistence変数をtrueに変更し各フレーム間で画面を自動的にクリアする様に変更します。
参考に図1プログラムをHEXファイルに変換し、それをSimbuino4Webでエミュレートした結果を図2に示します。

#include <SPI.h>#include <Gamebuino.h> Gamebuino gb; #include <EEPROM.h> #include <avr/pgmspace.h> #define SX 64 #define SY 32 byte buffer[SX * SY / 8]; unsigned int pop = 0; unsigned int prevPop = 0; byte resetCounter = 0; unsigned int gen = 0; byte graphCursor = 0; void setup() { gb.begin(); gb.titleScreen(F("Conway's game of life")); gb.pickRandomSeed(); reset(); } void loop() { if (gb.update()) { if (gb.buttons.pressed(BTN_C)) { gb.titleScreen(F("Conway's game of life")); reset(); } gen++; for (byte x = 0; x < SX; x++) { for (byte y = 0; y < SY; y++) { byte count = 0; if (getCell(x - 1, (y - 1 + SY) % SY)) count++; if (getCell(x, (y - 1 + SY) % SY)) count++; if (getCell((x + 1) % SX, (y - 1 + SY) % SY)) count++; if (getCell((x + 1) % SX, y)) count++; if (getCell((x + 1) % SX, (y + 1) % SY)) count++; if (getCell(x, (y + 1) % SY)) count++; if (getCell((x - 1 + SX) % SX, (y + 1) % SY)) count++; if (getCell((x - 1 + SX) % SX, y)) count++; byte cell = getCell(x, y); if ( cell ) { //if there is a cell if ( (count < 2) || (count > 3) ) { //and more than 3 neighbors or less than 2 gb.display.setColor(WHITE); gb.display.drawPixel(x + 1, y + 1); gb.display.setColor(BLACK, WHITE); pop--; } } else { //if there is no cell if (count == 3) { //and 3 neighbors gb.display.drawPixel(x + 1, y + 1); pop++; } else { //randomly add new cells if there is enough light if ((count == 2) && (gb.backlight.ambientLight > 950) && !(random() % 64)) { gb.display.drawPixel(x + 1, y + 1); pop++; } else { if ((count == 2) && (gb.backlight.ambientLight > 900) && !(random() % 128)) { gb.display.drawPixel(x + 1, y + 1); pop++; } } } } } } for (byte x = 0; x < SX; x++) { for (byte y = 0; y < SY; y++) { setCell(x, y, gb.display.getPixel(x + 1, y + 1)); } } if (gb.frameCount % 6) { if (prevPop == pop) { resetCounter++; if (resetCounter > 4) { gb.display.setColor(BLACK, WHITE); gb.display.cursorX = 38; gb.display.cursorY = 36; gb.display.print("Gimme"); gb.display.cursorX = 38; gb.display.cursorY = 36 + gb.display.fontHeight; gb.display.print("light!"); } } else { resetCounter = 0; } prevPop = pop; } gb.display.textWrap = false; gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.cursorY = 2; gb.display.println(F("Pop")); gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.print(pop); gb.display.println(" "); gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.cursorY += 2; gb.display.println(F("Gen")); gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.println(gen); gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.cursorY += 2; gb.display.println(F("Sun")); gb.display.cursorX = LCDWIDTH - 4 * gb.display.fontWidth; gb.display.print(F("\17")); //sun logo if (gb.backlight.ambientLight > 900) gb.display.print(F("\17")); else gb.display.print(F(" ")); if (gb.backlight.ambientLight > 950) gb.display.print(F("\17")); else gb.display.print(F(" ")); gb.display.setColor(WHITE); gb.display.drawFastVLine(graphCursor, 34, 14); gb.display.setColor(BLACK, WHITE); gb.display.drawFastVLine(graphCursor + 1, 34, 14); gb.display.drawPixel(graphCursor, LCDHEIGHT - (pop / 32) % 16); graphCursor++; if (graphCursor > SX) graphCursor = 0; } } void setCell(byte x, byte y, boolean state) { if (state) buffer[x + (y / 8) * SX] |= _BV(y % 8); else buffer[x + (y / 8) * SX] &= ~_BV(y % 8); } byte getCell(byte x, byte y) { return (buffer[x + (y / 8) * SX] >> (y % 8)) & 0x1; } void reset() { gb.battery.show = false; gb.display.persistence = true; pop = 0; gen = 0; graphCursor = 0; gb.display.clear(); gb.display.drawRect(0, 0, SX + 2, SY + 2); for (byte x = 0; x < SX; x++) { for (byte y = 0; y < SY; y++) { if (!(random() % 4)) { setCell(x, y, true); gb.display.drawPixel(x + 1, y + 1); pop++; } } gb.display.update(); } }
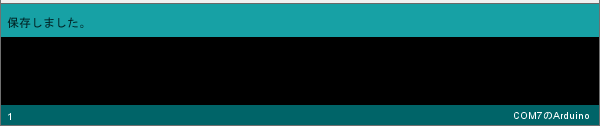
図1:プログラム例
■構文
gb.display.persistence = true or false
■パラメータ
true:各フレーム間の表示をクリアしない
false:各フレーム間の表示を消去する
■戻り値
デフォルト値はfalseです。
励みになりますのでよければクリック下さい(^o^)/